The test is open-everything with the sole limitation that you
neither solicit nor give help while the exam is in progress.
Pretend this is a real exam. Happy practicing!
- Solve for $x$. No calculators.
Solve exactly. Show your work.
$$ 3^{\log_2(\log_5{x})} = \left(\frac{6}{\log_2{5}}\right)^{\log_2{3}} $$
- Give the complexity function $T$
for the following code fragment, both as (a) a recurrence relation
and (b) in closed form using $\Theta$-notation.
public static long f(List a) {
if (a.size() > 1) {
f(a.subList(0, a.size()/2));
f(a.subList(3*a.size()/8, 7*a.size()/8));
f(a.subList(a.size()/4, 3*a.size()/4));
f(a.subList(a.size()/8, 5*a.size()/8));
f(a.subList(a.size()/2, a.size()));
for (int i = 0; i < a.size(); i++) {
for (int j = 0; j < i; j++) {
System.out.print("*");
}
}
}
}
- Sometimes you will see
the complexity function $\widetilde{O}$ — it is defined like this:
$$ \widetilde{O}(f(n)) =_{def} O(f(n)(\log{n})^k)$$
for some positive $k$. When an algorithm is known to have a complexity
function in $\widetilde{O}(n^3)$, for example,
some people will just say instead that it is in
$\widetilde{O}(n^{3+\varepsilon})$ — where, of course,
$\varepsilon$ is understood to be some arbitrarily small positive constant.
Why is this okay?
- Generate the ciphertext for the message
"woohoo" using the bifid algorithm with key "THEQUICKBROWNFOX". Lay out
the key row by row, left to right within each row, as is the convention.
Show the entire derivation.
- A binary min-heap starts off empty.
Show its shape (actually draw the tree) after each of the steps in the
following sequence. By min-heap we meam smaller values should appear on top.
- Insert 10
- Insert 4
- Insert 16
- Insert 3
- Insert 1
- Remove
- Insert 7
- Remove
- Remove
- Remove
- For the following graph:
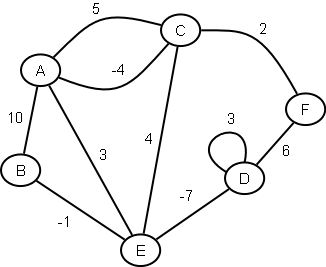
- How many blocks are there?
- Give a Depth First Traversal.
- Give a Breadth First Traversal.
- Give a Minimum Spanning Tree.
- Can Dijkstra's algorithm be used to find a shortest path from $F$ to $B$?
- Give the shortest path from $F$ to $B$.
- Is this graph simple? Why or why not?
- Which edges are bridges?
- Which nodes are articulation points?
- Is there a Hamilton cycle? If so, name one. If not, why not?
- Identify a trail (any trail).
- Is there an Euler tour? If so, name one. If not, why not?
- Give a smallest vertex cover.
- What is the degree of node $D$?
- How many isolated nodes are there?
- Is the graph complete?
- Extra credit: What is the chromatic number of this graph?
- Extra credit: What is the maximum flow of this graph?
- Show how to find, in polynomial
time, the smallest independent set that is also a vertex cover.
- Determining whether a simple,
undirected graph is 3-colorable is NP-complete, but determining
whether it is 2-colorable is in P. Give a $\Theta(e)$
algorithm for determining 2-colorability.
- Is bogosort an example of a Las Vegas or Monte Carlo algorithm?
- Fill out the bottom-up dynamic programming table for the 0-1 knapsack problem given
a 15kg sack and the following items, processing them alphabetical order. (Note:
the table is sideways to fit on the page, so you will be filling it out column
by column.)
| A weight=4 value=13
| B weight=6 value=10
| C weight=5 value=8
| D weight=7 value=5
| E weight=3 value=14
|
---|
1 | | | | |
|
---|
2 | | | | |
|
---|
3 | | | | |
|
---|
4 | | | | |
|
---|
5 | | | | |
|
---|
6 | | | | |
|
---|
7 | | | | |
|
---|
8 | | | | |
|
---|
9 | | | | |
|
---|
10 | | | | |
|
---|
11 | | | | |
|
---|
12 | | | | |
|
---|
13 | | | | |
|
---|
14 | | | | |
|
---|
15 | | | | |
|
---|