- Given a window bordered by (1,2) at the lower left and (16,12) at
the upper right, give the screen coordinates of a triangle with
vertices (3,2), (10,7.5) and (5,5) when mapped into a viewport
with corners (100,100) and (400,200). Provide accurate illustrations
of the window, viewport, and the untransformed and transformed
triangles with your answer. Remember in screen coordinates y
increases as you go down.
- Describe the effect of the scaling transformation S(-1,1).
- Give the series of basic transformation matricies that transform
the triangle with vertices A = (3,-3), B = (7,-3) and C = (5,3) into
the triangle A' = (1,-2), B' = (1,-4) and C' = (-5,-3). Ensure that
A maps to A', B maps to B' and C maps to C'.
- Compute the midpoint of the Bezier Curve with control points
p0 = (0,0,1), p1 = (1,0,1) and p2
= (1,2,0).
- Given a 25cm x 20cm display operating in 1024 x 768 x 16 color mode
which is refreshed 30 times per second, and for which 10% of the
refresh cycle is spent in retrace, calculate
- the pixel aspect ratio,
- the size of the frame buffer, and
- the required data transfer rate in kilobytes per second.
- Let P = (2, 0, -5) and Q = (3, 3, 4). Suppose the projection matrix
has been modified with
glLoadIdentity();
gluPerspective(90.0, 1.0, 2.0, 10.0);
and that the modelview matrix is unchanged from its default
setting. To which points (in normalized device coordinates) are P
and Q transformed? Show your work by first indicating the frustum
produced by the gluPerspective()
call.
- Compute the midpoint of the Bezier Curve with control points
p0 = (0,1,1), p1 = (2,3,1), p2 = (1,0,3)
and p3 = (1,1,4).
- What is the size of a pixel on a 21-inch diagonal screen with physical aspect
ratio 8:5 operating in 1152 x 800 mode?
- A raster display system operating in 1280 x 1024 mode displays
60 frames per second, and 8% of its operating time is spent in
retrace. In what amount of time must the video controller be able
to write a pixel to the screen?
- We are designing a software module for computer-aided design in land
surveying. The world-coordinate database contains objects in a
two-dimensional world whose units are feet by feet. The display
screen has size W inches wide by H inches high, and pixel resolution
1152 x 900, and as usual, (0,0) is the upper-left corner. The user
window on the world is specified by a 3-tuple (xc, yc, S) where
(xc,yc) = the world coordinate point of the window center, and
S = the display "scale factor" in feet per inches, e.g. S = 50 means
that one inch on the display represents 50 feet in the world.
Write a function to map a world point to a device point, in your
favorite programming language.
- Recall that we have performed simple animations with pixmaps by first
ANDing a mask to the screen and then ORing the pixmap. What happens
if we replace the OR with an XOR?
- Given a window with center (25,99) and a 322mm x 199mm screen with
resolution 1024x1024, map the world point (38,84) into screen
coordinates, where the viewport is (10,10)-(883,492), assuming 1mm
(1 unit) in the world is 4mm on the screen.
- Write a function to compute the perimiter of a polygon represented
by a circluar linked list.
- Prove or disprove: A × (B × C) = (A × B) ×
(A × C).
- Give the set of affine transformations that the describe the following
fractal. Also give the fractal dimension of this figure.
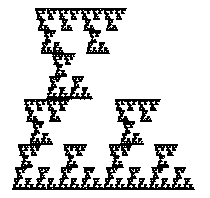
- What is the functional (parametric) form of the line passing through
(1,4,-3) and (-2,1,7)?
- How can a light pen be used as a valuator? A joyswitch as a string?
A speech recognizer as a choice?
- Discuss the advantages and disadvantages of performing two-dimensional
clipping in world-coordinates (before transformation) and performing
clipping in device-coordinates (after transformation).
- Illustrate the best-case and worst-case situations for line clipping
using (a) the Cohen-Sutherland algorithm and (b) the Liang-Barsky
algorithm.
- Recall that the Triadic von Koch Curve is produced by the L-System
Axiom F
Rule F → F+F--F+F
Angle Pi/3
Consider the family of curves {C(a) | 0 <= a < = pi} with the same
Axiom and Rule but with variable angle a.
- Give the fractal dimension of the curve C(a), as a function of a,
of course.
- Use your answer in part (a) to compute the dimension of the curves
C(0), C(pi/2), and C(pi). Explain these results geometrically using
sketches of the first few steps in the developments of each of these
curves.
- Give an (optimized) function to compute the reflection of one vector
about another, assuming both vectors are already normalized.
- Explain the visual effect that occurs when during animation of a
Gouraud shading polyhedron, the center of a highlight moves from
one vertex to another along an edge.
- Explain the essential difference between the "Scan-Line" hidden surface
removal algorithm and the multiple Z-buffer technique which uses a
buffer for each scan-line. lllustrate with pseudo-code.
- Defend or discredit the following statement: "The running time of the
Z-buffer algorithm for n polygons is just a little bit longer than that
of the algortihm which simply draws the n polygons, because at each
pixel you do only one comparison which takes almost no time at all."
- A raster video controller has an 18-bit DAC and comes equipped with
768K of video RAM.
a) What is the maximum display resolution (at 4:3) you
can provide in a 256 color mode?
b) In a 640 x 400 x 16 color mode, how many pages can be stored?
c) How many bytes are required for a lookup table in an 8-page
320 x 200 mode?
- What is the pixel ratio of a standard display operating in "EGA"
mode (640 x 350)?
- How long would it take to load a frame buffer for a 1024 x 768 x 16
mode given that we can transfer 100,000 bytes per second?
- Give the window-to-viewport transformation that maps a window centered
at (0,0) to a 19-inch diagonal screen of resolution 1152×900 in such
a way that the area of images in the viewport is twice that of the
objects from which they were produced. The viewport is the lower-right
fourth of the screen. The viewing area of this monitor is three-fourths
as tall as it is wide.
- Design an Ada package, C++ class, ML structure, CLU custer, Modula
module, Java class, or Object Pascal unit for rendering with multiple
light sources. Give a complete interface, and provide a sketch of the
body, including at a minimum the body of the function computing the
intensity.
- Explain the shape of J(λz.z2-2).
- Write some code that pans while the left mouse button is
held down.
- For each of the following implementations of rubberband line drawing,
describe a situation in which the interaction appears "invisible"
or explain why the method never produces an invisible interaction:
a) Xoring the line color into the frame buffer.
b) Complementing the pixels in the frame buffer.
c) Saving the pixels in the frame buffer and displaying the rubbery
lines in the actual line color.
- Give a specific example of a line that is clipped incorrectly in
3-D screen coordinates. That is, choose specific values for all
of the 3-D viewing parameters and compute the transformation of
a line segment that extends from inside the view volume to a point
behind the eye point. Provide an illustration that shows that
clipping this transformed line is not the same as clipping the
original line and then transforming to screen coordinates.
- A video controller comes equipped with 2MB of video RAM and 6-bit
registers for red and green color generation and a 4-bit register for
blue. What is a good resolution to use in a single-page full color mode?
How many pages could you have in a 640×480, 64-color mode?
How many bytes would you need for a lookup table in a 32-page
512×384 mode?
- Why in practice does the running time for the z-buffer algorithm remain
nearly constant as the number of polygons increases?
- Show how, in a ray tracing program, to compute successive rays across
a scan-line incrementally.
- Write a Java component that displays a circle icon
and notifies its listeners of the angle "selected" from it when
the mouse is pressed.
- Write a function to draw a 6cm square centered on the screen.
- Give the functional form of the line passing through (2,0,3) which is
perpendicular to the line connecting (1,1,3) and (4,2,6).
- Give the two-dimensional transformation for reflection about the
line λu.(u,-u), arriving at your answer
solely by intuition or a simple sketch. Show that this is equivalent
to rotation by -45 degrees followed by reflection about the y-axis
followed by rotation by 45 degrees.
- A display system comes with 4M for video storage and a 32-bit DAC.
What is the best resolution obtainable without lookup tables?
How many pages can we have in a 800 x 600 x 256 mode?
How large of a lookup table is required for a 4-page 1280 x 1024 mode?
- As you know all drawing that is done to a raster CRT is ultimately
done by loading pixel values into a frame buffer. Suppose you
had a linear-mapped frame buffer of size 800 x 600 x 256 colors,
starting at memory location FRAME_BUFFER_START.
Suppose you also had the C++ function
void fillMemory(byte* startAddress, int numBytesToWrite, byte value)
which fills all numBytesToWrite
bytes starting at memory location
startAddress
with value
. Implement the C++ function
void fillRectangle(int x1, int y1, int x2, int y2, byte color)
using only fillMemory()
calls. Do not forget to
"clip" and do not assume the corner coordinates are sorted.
If you don't know C++ very well, rephrase the problem in terms
of Java where the frame buffer is a big one-dimensional array of
48000 bytes.
- Why is not possible in general, to dim a portion of a screen in
indirect (indexed) color mode? Sketch an algorithm that provides
fairly decent approximations in all cases.
- Write a function to determine whether two vectors are anti-parallel,
without using division.
- A problem that often arises in scan-conversion is writing a single
pixel twice. For example in outlining polygons, pixels may be written
twice if we simply scan convert each edge. Why might we want to
avoid this situation?
- Recall that an affine transformation is a function
f(x,y) = (ax+by+e, cx+dy+f)
where a, b, c, d, e and f are constants and ad-bc!=0. The reason
for the last requirement is that when ad = bc then the matrix has
no inverse, as you can see by looking at the equation for the inverse.
Explain geometrically why the inverse does not exist.
- Why is it that writing certain applications using concurrent techniques
like threads and tasks still often a good idea even if tasking is
cooperative? Support your answer with a very brief sketch of an example
such as a simulation of molecules bouncing around in a volume, or
drawing Sierpinski triangles, or something like that.
- Give the matrix which transforms points in eye coordinates to screen
coordinates, for the situation in which the eye is located at the point
(0, 0, k), the front clipping plane at n = k and the back clipping plane
is n = infinity. What is wrong? Give a geometric interpretation of
why this situation is problematic.
- An ellipse in two-dimensional space is a set of points (x,y) satisfying
ax2 + by2 + c = 0 for constants a, b, and c satisfying
certain conditions.
a) What conditions must be placed on a, b and c to make the definition
precise?
b) Prove that a circle centered on the origin becomes and ellipse when
transformed by S(2,1).
- The quantity "cos(phi)" in the simple shading model can be approximated
by the cosine of the angle between N and the average of L and V; this
angle is sometimes called beta. The idea is that this approximation
saves us the trouble of computing R.
a) Do we really save a lot of time? Count additions, multiplications,
etc.
b) Express beta in terms of phi, assuming L, N and V are coplanar.
c) How large does phi have to be before the error in the approximation
is over 10%. Over 40%?
- What happens when you ray trace a picture and the shininess exponent
becomes "way too large"? How can you alleviate this problem?
- Show formally how to compute the hit time of an arbitrary ray with
the primitive cone.
- The figure ABCD where A=(-2,0), B=(0,-2), C=(-2,-4) and D=(-4,-2)
can be transformed into A'B'C'D' where A'=(1,-1), B'=(3,3), C'=(6,3)
and D'=(4,-1) by the composition of simple transforms T2*H1*S1*R1*T1.
Give the matrix form of these five transformations. Then express
the composite transform using only one scale, one rotation and one
translation.
- Prove or disprove that two successive rotations are commutative.
- For the following fractal, give its fractal dimension, and an
L-system to describe it.
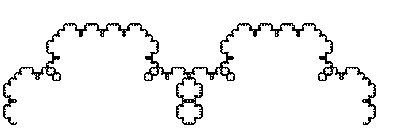
- To what point is (7,8) transformed when the coordinate system is rotated
82 degrees counterclockwise?
- Give the transformation that maps the square (0,0)-(1,0)-(1,1)-(0,1)
into the trapezoid (0,0)-(1,0)-(0.75,1)-(0.25,1).
- Write a program that illustrates the operation of the rocket launcher
in the old Space Invaders game. That is, allow the user to move a
launcher along the bottom of a screen or window and fire rockets
with left-button clicks. If the left button is held down, put
the launcher in "rapid-fire" mode. If you have time, add a single
row of invaders that move back and forth across the top of window
but don't fire at you.
- Write a program that does a cool lookup table animation. Do not
perform boring animations like bouncing balls or marquees.
- The orbit of a transform T beginning at point p0 is
the sequence of points p0, p1, p2,
p3,... where pi+1 = T(pi).
Given
type Transform = Point -> Point;
val Plot: Point -> unit;
write an ML function to plot orbits.
- Write a procedure to display the result of applying to the polygon
(-0.4,-0.4)--(0.7,-0.4)--(0.7,0.2)--(0.15,1.0)--(-0.4,0.2)
the transformation
λ(x,y).(x/2 + x*x/1.8 + y/5 + 0.3, y/2 + x*y/1.7 + 0.3)
Make sure your result is accurate to within the pixel resolution.
- Make a neat C++ class for two-dimensional affine transformations.
The component should support translation, scaling, rotation, shear
and reflection: overload the function call operator to apply
transformations to both points and polygons (polygons can simply
be lists of points). Also, you must support the composition of
transformations: overload the multiplication operator for this.
- Build a COM component for two-dimensional affine transformations.
The component should support translation, scaling, rotation, shear
and reflection.
- Give a sequence of basic affine transformations that transform
the following wedge into the extrusion of the right triangle with
vertices (0,0,0), (1,0,0) and (0,1,0) 10 units along the positive
z-axis.
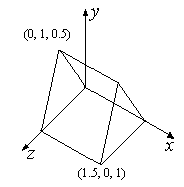
- Write a program to draw random hypocycloids.
- Write a function to determine whether a given two-dimensional
affine transformation is contractive. An affine transformation A is
contractive if and only if for all points P and Q, such that P is
not equal to Q, Length(PQ) > Length(A(P)A(Q)). In other words,
for all non-zero line segments L, L is strictly longer than A(L).
- Write a program to "draw" L-Systems.
- Plug in the definition of the Henon attractor into my orbit drawing
application and draw it.
- Write a program that draws random circles in the left side of the
window on one thread, and random rectangles in the right side on
another thread. You don't need to worry about thread synchronization
for this problem.
- Suppose we had a triangle with vertices (-1,-1,1), (0,1,1) and
(1,-1,1), and we had specified glFrustum(-5, 5, -5, 5, -2, 10) for
the projection matrix and were using only the default modelview
matrix. Sketch how the triangle appears on the display and explain
why the display looks like it does.
- A video card comes with 4MB of memory and has the customary
24-bit DAC.
a) What is the maximum resolution using a 4:3 pixel ratio that
you can obtain in direct (non-palettized) color mode?
b) What data transfer rate do you need to get pixels from the
frame buffer to the screen in this mode for 72 MHz operation?
c) How many 640 x 480 x 16-color pages could you pack into
the card?
d) If we wanted 6 pages of 1024 x 768 resolution, how many bytes
would we need for a color table?
- Design your own .IFS file. Make sure it looks really cool. Generate
a hard copy output of it.
- Design your own L-system. Make sure it looks really cool. (To be
really cool, it must use the '[' and ']' commands.) Generate a hard
copy of it, and a description of why it looks the way it does.
- Give the series of basic transformation matrices that transform
the wedge below so that it can sit "on top of" the following
parallelepiped to form a simple "house" with the ridge line of the
roof parallel to the z-axis. The height of the resulting house
should be 5.
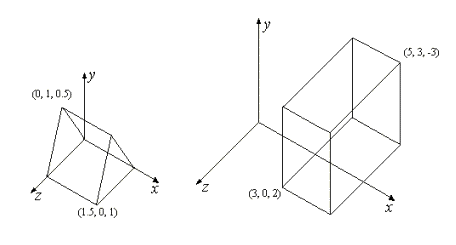
- Make a VRML world with animated rats running around a room.
- Modify my landscape class so that it can be lit with a spot
light source.
- Make a VRML house with a trap door in it.
- Write a Java application or applet to plot orbits. Make a beautiful
and sensible GUI, and architect it so that it works like the orbit
functions are "plugins" of some sort (classes loaded at run time
in response to selection from a list box or simply text strings
representing functions...).