-
let [x, y] = [0, 0];
console.log(93.8 * 2 ** x + y);
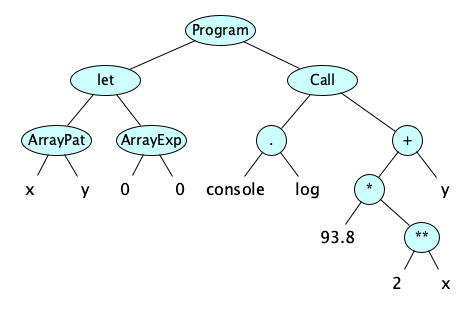
-
import x from "x"
console.log(93.8 * {x} << x.r[z])
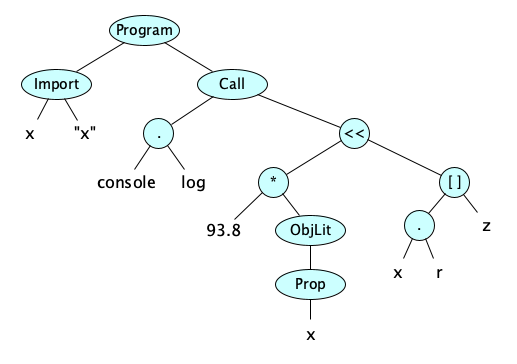
-
const x = x / {[x]: `${x}`}[x]("y")
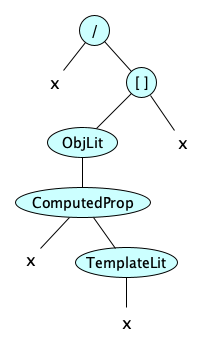
-
class C {
f({a, b: c}) {return ([a, f]) => C}
}
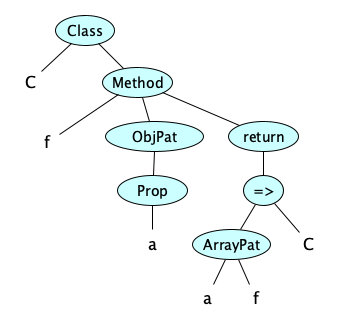
- To make the expression
-2**2
...
- ...evaluate to 4, we make negation have higher precedence than exponentiation:
Exp = Exp "+" Term | Term
Term = Term "*" Factor | Factor
Factor = Primary "**" Factor | Primary
Primary = "-" Primary | num | id | "(" Exp ")"
- ...evaluate to -4, we make negation have lower precedence than exponentiation. You can put the negation at the beginning of wither the
Exp
or the Term
rule:
Exp = "-"? (Exp "+" Term | Term)
Term = Term "*" Factor | Factor
Factor = Primary "**" Factor | Primary
Primary = num | id | "(" Exp ")"
- ...be a syntax error, we put negation and exponentiation in the same rule:
Exp = Exp "+" Term | Term
Term = Term "*" Factor | Factor
Factor = Primary "**" Factor | "-"? Primary
Primary = num | id | "(" Exp ")"
- Here is a grammar for Astro++ that enforces the appearance of break statements only within while loops. (In practice, most people don't actually capture this in the grammar, but it's a good exercise to actually do it!)
Astro {
Program = StmtNoBreak+
StmtNoBreak = Assignment
| PrintStmt
| IfStmtNoBreak
| WhileStmt
Stmt = Assignment
| PrintStmt
| IfStmt
| WhileStmt
| BreakStmt
Assignment = id "=" Exp ";"
PrintStmt = print Exp ";"
BreakStmt = break ";"
WhileStmt = while Exp Block
IfStmtNoBreak = if Exp BlockNoBreak (else (BlockNoBreak|IfStmtNoBreak))?
IfStmt = if Exp Block (else (Block|IfStmt))?
BlockNoBreak = "{" StmtNoBreak* "}"
Block = "{" Stmt* "}"
Exp = Exp1 ("<="|"<"|"=="|"!="|">="|">") Exp1 --relational
| Exp1
Exp1 = Exp1 ("+" | "-") Term --additive
| Term
Term = Term ("*" | "/" | "%") Factor --multiplicative
| Factor
Factor = Primary "**" Factor --exponentiation
| "-" Primary --negation
| Primary
Primary = id "(" ListOf<Exp, ","> ")" --call
| numeral --num
| id --id
| "(" Exp ")" --parens
numeral = digit+ ("." digit+)? (("E" | "e") ("+" | "-")? digit+)?
print = "print" ~idchar
while = "while" ~idchar
if = "if" ~idchar
else = "else" ~idchar
break = "break" ~idchar
keyword = print | while | if | else | break
idchar = letter | digit | "_"
id = ~keyword letter idchar*
space += "//" (~"\n" any)* --comment
}