Web Applications
Here is a little introduction to webapps.
What is a Webapp?
Definition
Loosely speaking, a web application is a program that runs on a computer with a web server and is accessed via a web browser (the “client”) that communicates with the server via the HTTP or HTTPS protocol.
In HTTP(S), the client sends a request to the server and the server replies with a response:
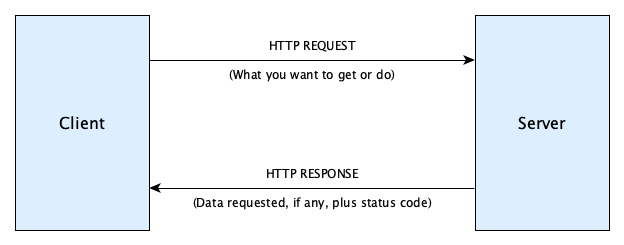
If the server always sends back the same data in the form of HTML, CSS, images, videos, and similar resources without any user interaction, we call the app a web site.
If there is user interaction (mouse clicks, tapping and swiping, phone shaking, keypresses, etc.) that affect the execution of the program, we have a web app.
If the server only produces raw data (generally in text or JSON), then we speak of a web service.
Check out the full Wikipedia articles on
web applications,
web servers, and
web services.
Examples
Strawberry Pop-Tart Blow-Torches is a web site. It’s just HTML with images. Last updated in 1994. Looks great.
Gmail is a web app. All you need to use it is a web browser. You login, create and organize filters, read messages, reply, forward, send, and delete, and logout. Messages exist in a data store on the server, as does all the code to generate pages. Of course the “pages” include a fair number of scripts that the browser knows how to execute, but these scripts are kept on the server and downloaded on demand.
The Poké API is a web service. Enter https://pokeapi.co/api/v2/pokemon/ditto
into your browser’s address bar. You got back pure data.
Web Applications vs. Native Applications
With webapps, you do not have to package up software for distribution and installation on client computers. Updating the software is easier too, since you don't have to ship an update and hope users know how do install it. You just make the change on the server yourself, and users see the new version the next time they visit your site (though some browsers cache old pages a bit too aggressively).
Writing and Deploying Simple Web Apps
If your app is very simple, you can let your web browser take care of all of the details of the HTTP protocol so you don’t have to be concerned with it at all!
You will just create (1) HTML to describe your app’s content; (2) CSS to describe your app’s presentation, and (3) JavaScript, to program the app’s interactivity. You may also include (4) images, video, text, and other information. These resources are all placed on the server, and the clients will use a web browser to fetch and render them.
Now where can you host your web app? That is, where is your web server? You can get your own server of course, or use one provided by a service. Services for simple web apps include
The p5js Web Editor,
Replit,
Glitch, and
Code Sandbox.
Here is a code sandbox for a simple webapp:
CLASSWORK
We are going to look at each of the files in the web app and discuss them in class.
Each of the services listed above will host your webapps! For Code Sandbox, you’ll see the deployment url right above your preview window. The sandbox above is hosted at https://g83hi.csb.app/.
CLASSWORK
We’ll do a code-along here so you can write and deploy your first webapp!
Web Apps IRL
As we scale up a little from the introductory web apps that place all the HTML, CSS, and (very minimal) JavaScript on a single server, we’ll next deal with these two things:
You may have heard about APIs. These are services on the web providing data on such things as the weather, restaurants, recipes, spaceship locations, movies, and countless other things. Some APIs are free and some you have to pay for.
Now what about storage? There’s one thing you need to know about HTTP requests. They are stateless. They don’t remember anything. If you need to keep track of anything you have to store it. You can store information client-side within your browser’s localStorage or server-side in a database. Whichever way you choose to store data, you as a programmer are responsible for security. This is a big topic that we will cover later in the course.
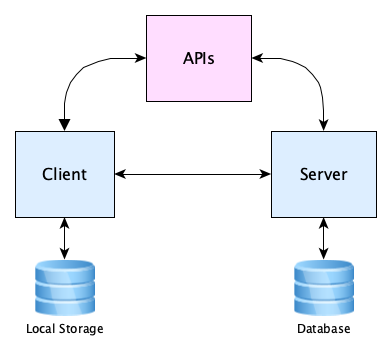
Here’s something with a bit more. Don’t worry, you don’t have to understand every piece of this right now:
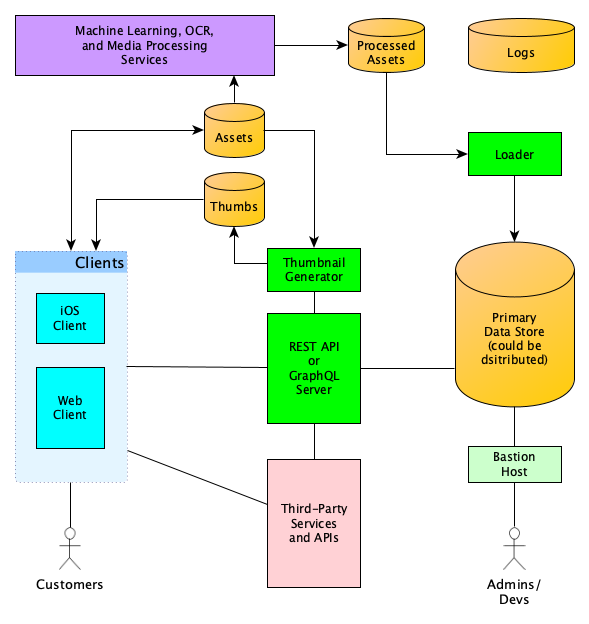
This shows that complex web apps have lots of different elements:
- Clients and servers, for the main work
- Databases, which store stuff that needs to be accessed very quickly
- Storage, for videos, images, and other big things
- Cloud Functions, which process events on the back end (behind the scenes)
- Logs
- And more
Here are discussion points for class:
- Although we generally use the term web app for browser-based apps, native mobile clients for iOS or Android can still make HTTP requests to a web server. So larger systems may provide web services for both web and mobile clients.
- The web client is a traditional webapp: it serves HTML and CSS and JavaScript.
- The web client generally does not talk to directly to the business data store; instead the gatekeeper to the business data is a web service itself. This server can expose a REST API or GraphQL service. It is often known as “the API.”
- The primary data store can be a relational database, a document database, a graph database, or maybe a set of different stores, including search indexes and maybe even data warehouses. The API abstracts away how the database is physically structured.
- The API generally supports CRUD (create, retrieve, update, delete) access to business resources.
- Certain API invocations can cause notifications, such as emails to be sent to customers. These can happen within the app backend itself or through a third-party email service.
- Clients can talk directly to asset (or media) storage. You’ll want to keep images, videos, and large documents in an asset store, not in your business database. Clients will generally ask the primary data store for a secure, time-bombed URL to access the asset store.
- Uploading large assets should trigger thumbnail generation to take place. Thumbnail images are then written to a second asset store just for thumbnails.
- Many assets will require additional processing like video encoding, image processing, optical character recognition, or analysis by machine learning software (for, say, feature detection). The results of such processing can be written to a holding area, and another process can pick data from this store and augment business data with this new information.
- Everything should be logged, except where logging would violate privacy or security concerns.
- Administrators and trusted developers may need, form time-to-time, to peek at the data store directly. They should never do this from their own machines; rather, they would tunnel into a bastion or jumpbox in the same security group as the data store. The backend provider might also expose a console or command line interface.
Exercise: For fun, look up the difference between a bastion and a jumpbox.
Backend as a Service
Believe it or not, here’s another set up:
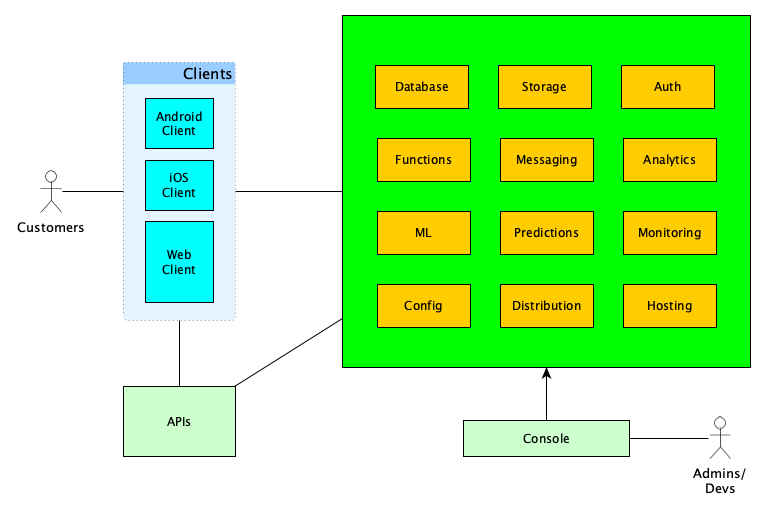
That’s right, the whole backend as one giant service, accessible via SDKs on the clients, or via APIs, or a console for admins.
One of the more popular such services for web apps is Firebase.
What’s Next?
The topic of web applications is extensive. The immediate next steps would be to go into more depth with HTML, CSS, JavaScript, and HTTP. Much more to follow after those topics, too!
Recall Practice
Here are some questions useful for your spaced repetition learning. Many of the answers are not found on this page. Some will have popped up in lecture. Others will require you to do your own research.
- Web apps run on a computer with a __________ and are accessed with a __________.
Web server, web browser.
- How does a web client communicate with a web server?
Via the HTTP (or HTTPS) protocol.
- In HTTP, clients send __________ to a server and the server sends __________ back to the client.
Requests, responses.
- What is a web site?
A collection of web pages that are “static” and do not require user interaction.
- What kind of user interactions are used in a web app?
Mouse clicks, tapping and swiping, phone shaking, keypresses, etc.
- What is a web service?
A web application that only produces raw data (generally in text or JSON).
- What are some examples of web apps?
Gmail, X, Instagram, etc.
- How do web apps differ from native apps?
Web apps run in a browser and do not need to be installed on a client computer.
- What are the three languages used in webapps?
HTML, CSS, JavaScript.
- In a web app, HTML is used for __________, CSS for __________, and JavaScript for __________.
Content, presentation, interactivity.
- What are some services that you can host your web apps on?
The p5js Web Editor, Replit, Glitch, Code Sandbox.
- What is meant by the fact that HTTP is a stateless protocol?
It doesn’t remember anything—every request is fresh. If you need to keep track of anything you have to store it.
- What is a (web) API?
A service on the web providing data such as the weather, restaurants, recipes, spaceship locations, movies, and countless other things.
- What kind of storage is used on the client (browser) side of a webapp?
localStorage.
- What kinds of storage are used on the server side of a webapp?
Databases mostly, but there’s also “Cloud Storage” for large media.
- What is the meaning of CRUD in the context of APIs?
Create, retrieve, update, delete.
- Developers and administrators needing access to data on the cloud should not connect their machines directly to cloud servers; instead, they should use a __________ or __________.
Bastion, jumpbox.
- What is a BaaS and what kinds of services does it provide?
Backend as a Service. It provides a complete backend for your app, including databases, storage, and more.
- What is the most well-known BaaS on the Google Cloud?
Firebase.
Summary
We’ve covered:
- A definition of the term webapp
- Differences between a webapp and a web service
- Differences between webapps and native apps
- A simple webapp architecture
- A more complex architecture
- Backend as a Service